Create your own mapping helper class
To create your own codelibrary with a list of methods you can easily access from xslt in Link this is the way to do it.
First you need to create a custom class library in .Net with the methods you wish to have easy accessible in XSLT.
This could be simple c# methods but it could also be more complex methods that access additional data from your internal systems using API access. Maybe you need to extract the quantity on stock of a given item or fetch additional customer data from CRM or other system.
The code for that could look like this:
using System.Text.RegularExpressions;
namespace Bizbrains.Link.Sample
{
public class MappingHelper
{
public static String NewGuid()
{
return Guid.NewGuid().ToString();
}
public static string RegexReplace(string input, string regex, string replacement)
{
return Regex.Replace(input, regex, replacement);
}
public static string StringSplit(string input, string separator, string index)
{
int i;
if (!Int32.TryParse(index, out i))
{
return "";
}
string[] split = input.Split(separator);
if (i >= split.Length)
{
return "";
}
return split[i];
}
}
}
When build to a dll you can upload it to Link artifact store.

The class is then assigned to a namespace in the XslTransform itinerary step like this:
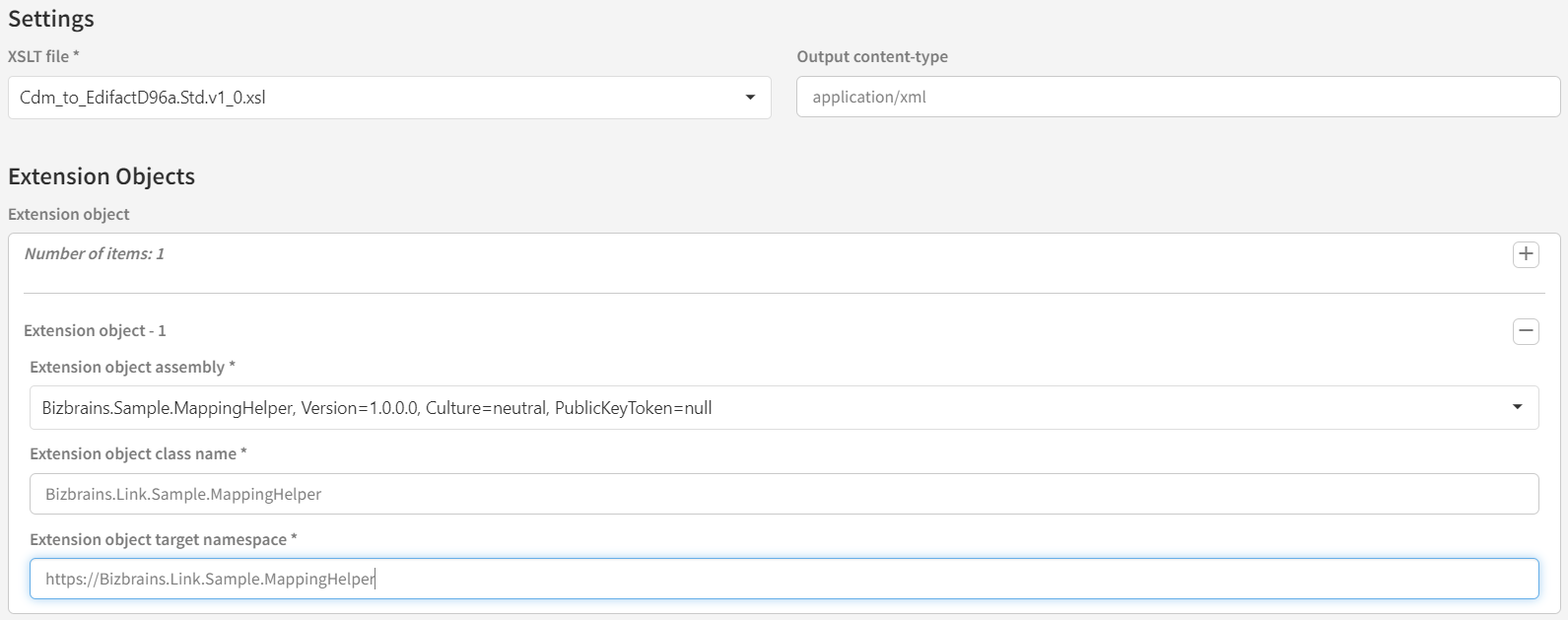
And all the methods could then be accessible from XSLT by reffering to this namespace with a prefix and access the methods from xslt with that prefix like e.g
<?xml version="1.0" encoding="utf-16"?>
<xsl:stylesheet xmlns:myHelper="https://Bizbrains.Link.Sample.MappingHelper" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:msxsl="urn:schemas-microsoft-com:xslt" exclude-result-prefixes="msxsl s0 myHelper" version="1.0" xmlns:s0="http://XsltProxyTest.Input" xmlns:ns0="http://XsltProxyTest.Output">
<xsl:output omit-xml-declaration="yes" method="xml" version="1.0"/>
<xsl:template match="/">
<xsl:apply-templates select="/s0:Root"/>
</xsl:template>
<xsl:template match="/s0:Root">
<ns0:Root>
<MyNewGuid>
<xsl:value-of select="myHelper:NewGuid"/>
</MyNewGuid>
</ns0:Root>
</xsl:template>
</xsl:stylesheet>
Accessing the Link API from a mapping helper class
If you have a mapping scenario where you need to include some data in the output that is not present in the input, but that is available in Link, you can use the Link API to look it up.
In order to access the Link API from a mapping helper class, you need to make the interfaces you need to use available to the class through dependency injection. This involves installing the necessary NuGet packages in the project of the helper class:
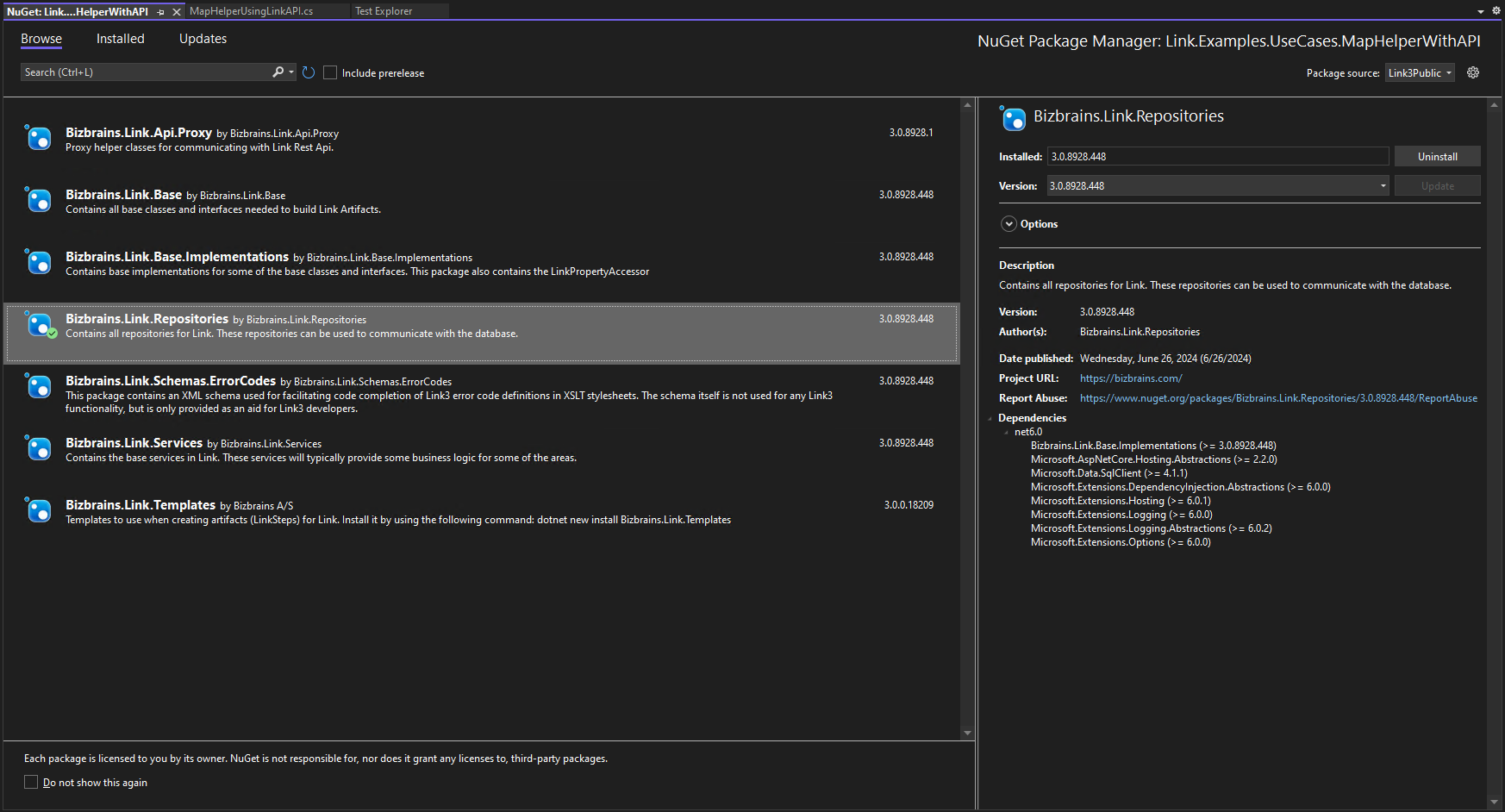
For instructions on how to access the Link3Public NuGet repository, please see this article.
The only packages you will typically need in this scenario are Bizbrains.Link.Repositories and/or Bizbrains.Link.Services.
IMPORTANT: Both repositories and services contain methods that change Link data in various ways, such as creating, updating or deleting entities. DO NOT use them in a map helper class. Only use methods that read data from Link. If you change Link data in a map helper class, it will be almost impossible for anybody to trace if there is a problem. If you absolutely need to change Link data as part of a flow, make a custom step to do it.
Here is an example of a map helper class which uses the Link API to look up the file name of the ingoing interchange of the document being mapped:
using Bizbrains.Link.Repositories.Transaction.Tracking;
namespace Link.Examples.UseCases.MapHelperWithAPI
{
/// <summary>
/// This example shows how to access the Link API from a map helper extension object.
///
/// DO NOT under any circumstances call API methods that actually make changes to the data in Link.
/// It will be impossible to trace if something goes wrong. Please only read Link data.
/// </summary>
public class MapHelperUsingLinkAPI
{
private readonly IInterchangeInRepository interchangeInRepository;
/// <summary>
/// In order to use the Link API in a class, the relevant interfaces (repositories and/or services)
/// must be added to the constructor like this.
/// </summary>
/// <param name="interchangeInRepository">Any number of repositories and services can be made available to the class in this manner.</param>
public MapHelperUsingLinkAPI(IInterchangeInRepository interchangeInRepository)
{
this.interchangeInRepository = interchangeInRepository;
}
/// <summary>
/// This method uses the InterchangeInRepository to look up the ingoing interchange
/// of the document being mapped and returns its file name.
///
/// NOTE: You cannot use async methods in a map helper class since the Xslt engine does not support it.
/// Since methods in the Link repositories and services are generally async methods, you need to call them
/// using .GetAwaiter().GetResult() as seen here.
/// </summary>
/// <param name="interchangeGuid">This is the interchange Guid of the ingoing interchange.
/// It can be looked up in the map using the LinkProxy method GetInterchangeInInterchangeGuid()</param>
/// <returns>The file name of the ingoing interchange, or an empty string if it cannot find the interchange.</returns>
public string GetInterchangeInFilenameByInterchangeGuid(string interchangeGuid)
{
Guid iGuid = new Guid(interchangeGuid);
var interchange = interchangeInRepository.GetByGuid(iGuid).GetAwaiter().GetResult();
if (interchange != null)
{
return interchange.FileName;
}
return "";
}
}
}
The example is also available in project form in the Link Documentation Examples repository.